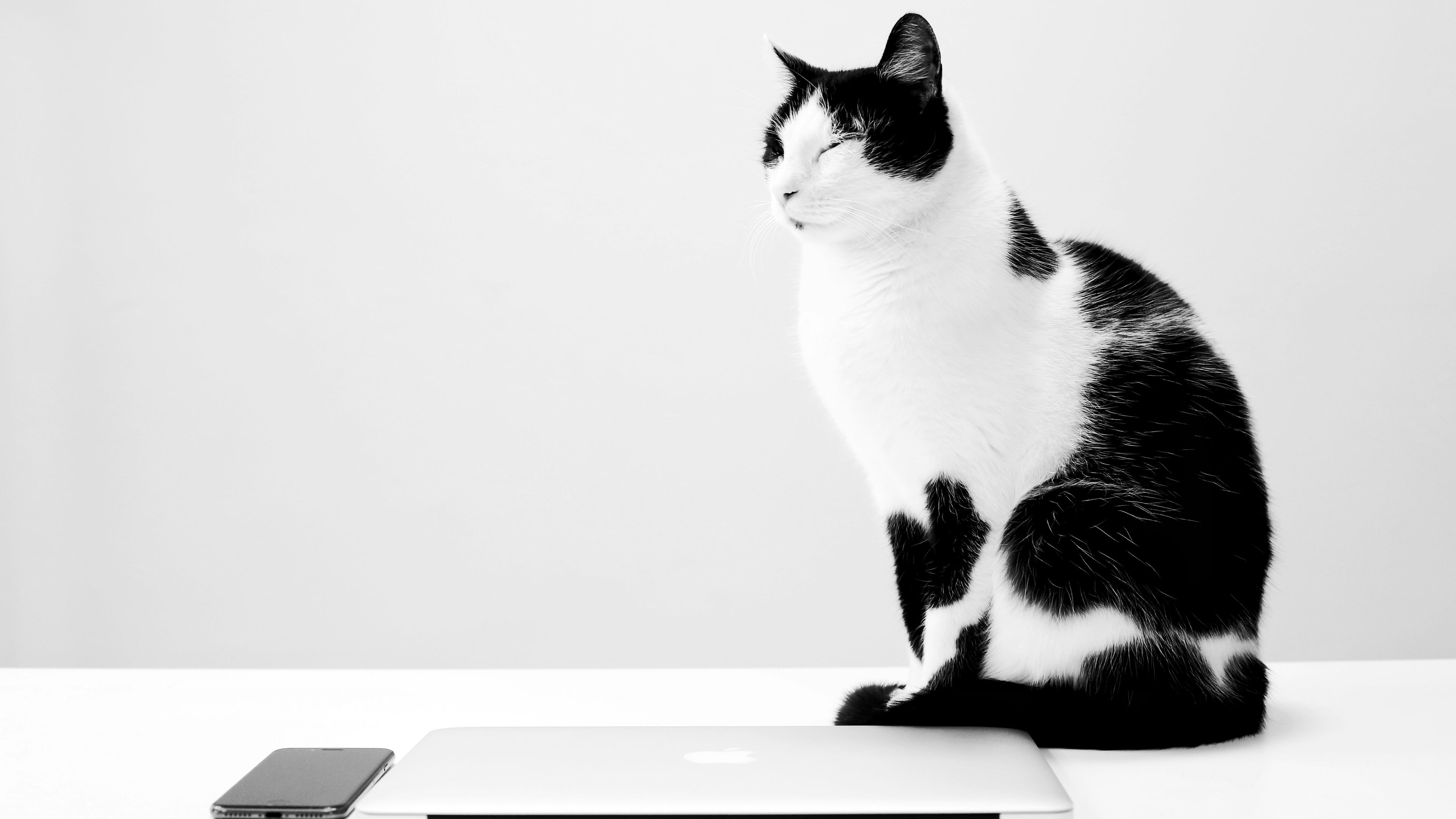
Developing your own website can be inexpensive with the main running cost being buying a domain, and of course your own time.
Using a "headless CMS" (content management system), such as Sanity, can provide great flexibility for your content across all sorts of devices and choice of modern frameworks including NextJS. Opposed to a traditional CMSs, a headless CMS can publish content to any "front-end" provider, enabling you to choose any sort of coding language and framework. There are many benefits including live editing & saving, image optimisation, generally quicker pagespeed loading, and TypeScript support (a file type that can point out errors during development mode) to name some.
NextJS is developed by Vercel, a popular 'Frontend Cloud' that simplifies deploying sites and integrates along well with Sanity.io and GitHub. The great thing about using such platforms is that they are open-source - having fairly generous free account plans and collaborative communities that are free to join.
All of this is to say that this guide has selected some of the more user-friendly tools out there that don't compromise on capabilities, customisation and scalability. Anyhow, if you're a hands-on person like me, let's dive into it, and do note that the tips throughout are geared for Mac OS users.
Resources needed prior
As mentioned before, you won't be spending out-of-pocket in this walkthrough other than buying a domain. While some of the platforms have trial account plans, after they expire you will be able to stay on fairly generous free plans to keep your site running. Prep on your desktop the following by installing and creating accounts etc to make the process smoother:
- NPM and node.JS (recommended to install the LTS version which includes npm)
- Visual Studio Code (note Visual Studio Code is different from Visual Studio)
- Check that you give Full Disk Access in your System Settings > Privacy & Security >
- Install recommended Extensions i.e. Node Essentials, Node Extension Pack, Tailwind CSS, TypeScript Debugger
- GitHub account
- GitHub Desktop
- Check that you give Full Disk Access in your System Settings > Privacy & Security >
- Sanity.io account (you can use your GitHub account as a login)
- There is a Slack community you can request to join for discussions & support
- Vercel account (you can use your GitHub account as a login)
- Cloudflare account (optional choice of domain provider)
- Buy an available domain from the Cloudflare Domain Registration. While this is an optional step, you may face issues using the Vercel link.
Jumpstart by working from a template
If you don't like the idea of starting from scratch and coding 101, you could quickly learn by working on a template and familiarising yourself with the architecture in a practical way. See this as a phase 1 for getting the bones of your site up and running. Then we'll continue in future guides how to further customise components, add layouts, CSS and install plugins and third-party tools like Google Analytics.
Create a Next-Sanity app via Vercel
In this guide, we're using https://vercel.com/templates/next.js/sanity-visual-editing-demo
1. Click the "Deploy" button and follow the instructions.
You'll be asked to log into your GitHub account. Then create and name a new "Git Repository". Choose something related to your desired domain name. Note that words should be separated by hyphens. Keep the "Create private Git Repository" ticked as you probably do want to keep your project private.
Add the Sanity integration and you'll be asked to sign into your account and create the new project.
Proceed to Deploy and let it run. Note the estimate build time might not be accurate. You can always open up a new tab to log into your Vercel account and check the progress.
2. Use GitHub Desktop to continue set up locally on your computer
In the GitHub Desktop, click on the Repository panel > Add > Clone Repository...
Select the project by name you created earlier via Vercel.
3. Ensure you have all Reading & Writing permissions
If you're a Mac OS user, use the shortcut (cmd + shift + F) from GitHub Desktop to "View the files of your repository in Finder".
At the top of the Finder window, click the 3 dots > Get Info
Then, in the popup, look towards the bottom for the "Sharing & Permissions:". Unlock the padlock to change the Privilege for your user profile to have "Read & Write" (if it doesn't already) and then click at the bottom the 3 dots > Apply to enclosed items > OK
and lock the padlock to save changes.
4. Open your project in Visual Studio Code
Back in GitHub Desktop you can use the shortcut (cmd + shift + A) to "Open the repository in your external editor".
5. Use Terminal to link up your project
Within Visual Studio Code open a new Terminal from the nav bar Terminal > New Terminal (or use the keyboard shortcut ctr + opt + `)
Copy + paste + Enter the follow to run as a command in Terminal:
npx vercel link
You'll be prompted to enter your Mac admin password to proceed.
Tip: whenever you get a Terminal error message about 'EACCES' you can prepend "sudo" to the command you want to run in Terminal.
Then follow the prompts to set up your Vercel project and link the project by the name you recently created.
When that's completed, copy + paste + Enter:
npx vercel env pull
6. Run your next.js project in dev mode locally on your computer
Next, in Terminal run the following:
npm install && npm run dev
This will install the necessary node.js modules etc and trigger dev mode. Meaning you'll be able to play around with your Sanity CMS in your browser your browser at http://localhost:3000/studio
and view the content at http://localhost:3000
Tip: you can hover on the url that appears in the Terminal messages and hold "cmd + click" to follow the link in your default browser.
You'll be able to access the localhost as long as the Terminal window is open. For future Terminal commands, open another Terminal window (keyboard shortcut: ctr + opt + `). Have a play around the CMS and create some test pages to familiarise yourself with the environment.
Tip: While Visual Code Studio extensions are great at highlighting errors, sometimes things get missed, so you can always double check your code by running in a new Terminal window the command:
npm run build
7. Remove the "Next steps" template block on the site
In Visual Studio Code, locate the "intro-template" folder and delete it.
Then locate the "Layout.tsx" file in components > shared >
Edit the file to remove instances of "IntroTemplate". You'll see the div class below the line for the footer to delete:
<IntroTemplate />
and the import call for that component at the top of the file to delete:
import IntroTemplate from 'intro-template'
8. Prepare your custom domain for publishing.
If you haven't already, register a domain, in this example via Cloudflare.
In your Vercel account online, go to your project's Settings > Domains
to add your domain. Follow the instructions to add your DNS records in your Cloudflare domain's DNS Records Configuration.
e.g. add Type "A" record for the nonWWW version of your domain and Type "CNAME" record for the WWW version of your Vercel domain url.
Tip: In the record settings, switch the Proxy status from "Proxied" to "DNS only"
Back in Vercel it will automatically validate configuration. Ensure that you haven't made "redirection loops" and that variations of the site domains point to the one desired domain version.
9. Deploy your site to your custom domain and GitHub repository
Go to your GitHub Desktop app to use the shortcut button "Commit to main". Best practice is to fill in a detailed Summary and Description of what changes you've made. For example, include a datestamp and list files you made changes to and the level of impact on your site.
This should trigger GitHub to "back up your copy of the site" and trigger Vercel to publish your site on your domain.
Congrats! In Vercel, you should be seeing the green Status "Ready" on your Production Deployment.
If not, you'll need to do some troubleshooting. You can click into your Vercel Deployment Details > Building > Errors to see the log of issues.
Or from your local project, use Terminal to run the command "npm run build".
Typical issues could be typos in the code - check for syntax errors, or your Vercel Project Settings > Node.js Version not matching what you've installed, or your Domains redirection settings.
You'll only need to make future "deployments" when making "backend" changes to, for example, page layouts and adding new components. You can always access your headless CMS from "yourdomain.co.uk/studio" and do content editing and publishing from there. You may need to wait a while for caching to clear before you see changes published. Make it a habit to hard refresh your browser (keyboard shortcut cmd + shift + R) before doing further troubleshooting. You can also force caching to clear from your Cloudflare account > Websites > Caching > Configuration > Purge Everything